
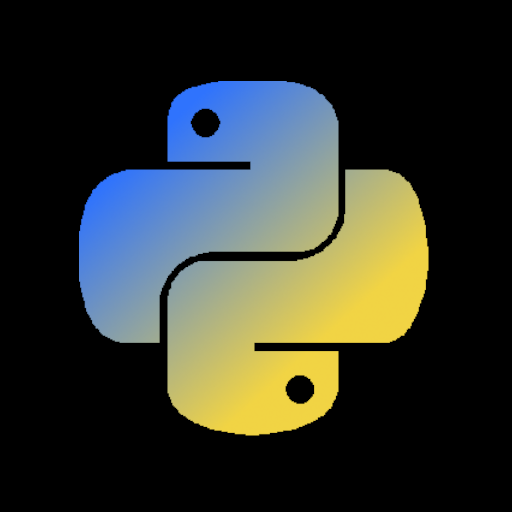
Well == is a question or a query rather than a declaration of the state of things because it isn’t necessarily true.
You can write
a = (3 == 4)
which is perfectly valid code; it will just set a
to be false
, because the answer to the question “does 3 equal 4?” is no.
I think you’ve got it anyway.
Yes:
Operator '+' cannot be applied to types 'number[]' and 'number[]'.
We’re talking about Typescript here. Also I did say that it has some big warts, but you can mostly avoid them with ESLint (and Typescript of course).
Let’s not pretend Python doesn’t have similar warts:
>>> x = -5 >>> y = -5 >>> x is y True >>> x = -6 >>> y = -6 >>> x is y False >>> x = -6; y = -6; x is y True
>>> isinstance(False, int) True
>>> [f() for f in [lambda: i for i in range(10)]] [9, 9, 9, 9, 9, 9, 9, 9, 9, 9]
There’s a whole very long list here. Don’t get be wrong, Python does a decent job of not being crazy. But so does Typescript+ESLint.
“It’s so bad I have resorted to using Docker whenever I use Python.”